Start with a new project
We’re going to build a Twitter style app, using Blazor Server.
We’ll keep our requirements dead simple so we can get something working, but also see how all the moving pieces fit together (and crucially how to separate your UI and Business Logic concerns, so you can easily swap out the UI layer at a future date).
Here are the two key features we’re going to build:
- Show a list of tweets
- Post a new tweet
Prerequisites
You’ll need the latest version of Visual Studio or Visual Studio Code and I think you can probably follow along if you’re using JetBrains Rider too.
Make sure you have the latest version of the .NET Core 5.x SDK installed. If you have VS 2019 or higher it should already be installed for you, if not, go grab it here.
We can’t get very far without a project so let’s create that first.
If you’re using Visual Studio, use the Blazor App project template…
If given the choice, make sure to choose “Blazor Server App” as the project type… and leave all the other options as they are (Configure for HTTPS, No Authentication etc).
You can also create this project via the command line…
dotnet new blazorserver
This will create the new project in whichever folder you’re in when you run the command…
I called my project “MyTweets”…
You should end up with a project which looks like this…
Run this now and you should get a Blazor app running in your browser (if you’ve been watching any Blazor videos recently you’ve almost certainly seen this demo app many times!).
What is Blazor really for?
If you’ve spent any time with ASP.NET (MVC, Razor Pages, even WebForms) you’re probably wondering where Blazor fits in.
For me I see Blazor as primarily about building the user interface for your application.
You can run Blazor in two different ways:
- In the browser using Blazor WebAssembly
- On the server using Blazor server
If you opt to use Blazor in the browser it acts a bit like React, Angular or any of the other javascript frontend frameworks. In this mode Blazor makes calls to a backend API to persist or fetch data.
With Blazor Server your Blazor app runs on the server, but sends messages to the browser so it knows how to update the DOM (to show/hide HTML elements and react to events like button clicks).
In both cases it really helps to think of your applications in two discreet parts:
- The UI (including presentation logic)
- Business logic
You will end up with some logic in the UI part of your application (Blazor components), but it’s presentation logic (show this, hide that, handle this button click).
Your business logic goes somewhere else.
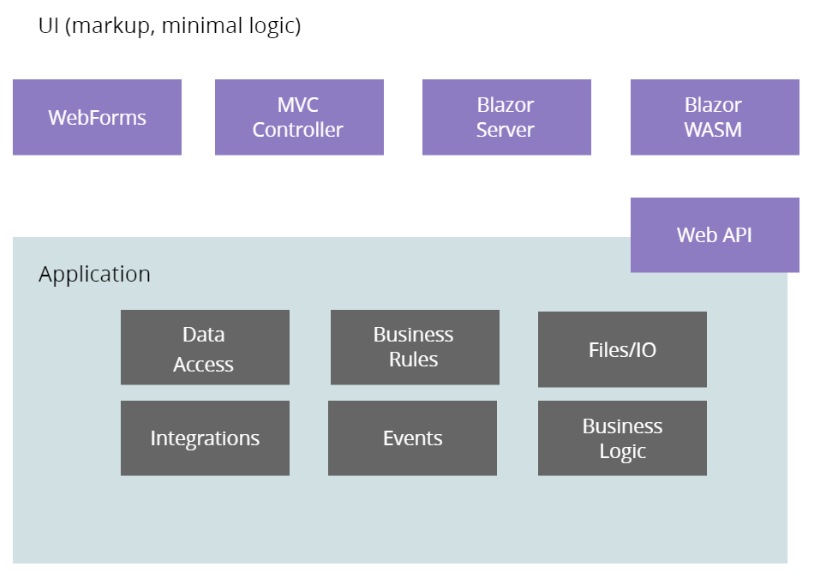
This separation makes your life a lot easier!
It means you’re able to try swapping out the frontend for your application (to Blazor, ASP.NET MVC, even React if you want to!) without changing how the core parts of your application work.
Here’s how we’ll approach the rest of this course:
The next lesson will start with the UI, building out a simple list of tweets using Blazor’s component model.
Then we’ll see how to build the key business logic (and persistence) in C#.
Finally we’ll hook the two up together.
Sound good? OK hit the link to continue to lesson two :)