Angular 2 and .NET Core – route directly to your components
Last time out, we took a quick look at creating a simple HelloWorld component and displayed it on one of our existing web pages using its selector.
<helloworld></helloworld>
Which rendered something like this…

Using the helloworld
selector you can now include this component anywhere you like in your app, it doesn’t matter how many times you use it. Anywhere you can write html, you can include your component; this includes in the template of another component. For example, you can display your helloworld
component in the template for the home
component.
<h1>Hello, world!</h1>
<helloworld></helloworld>
<p>Welcome to your new single-page application, built with:</p><ul> <li><a href='https://get.asp.net/'>ASP.NET Core</a> and <a href='https://msdn.microsoft.com/en-us/library/67ef8sbd.aspx'>C#</a> for cross-platform server-side code</li> <li><a href='https://angular.io/'>Angular 2</a> and <a href='http://www.typescriptlang.org/'>TypeScript</a> for client-side code</li> <li><a href='https://webpack.github.io/'>Webpack</a> for building and bundling client-side resources</li> <li><a href='http://getbootstrap.com/'>Bootstrap</a> for layout and styling</li></ul></pre>
Route to a component#
All well and good, but what if we want to show this content by itself, not nested in another page.
The Angular 2 router is a powerful tool which enables you to do just that. Specifically you can configure it to route to a component when the user enters a specific url for your site.
So in this case, we could configure it so that navigating to http://thissitesaddress.com/hello routes directly to the hello world component.
Open up your app.module.ts file and make the following simple change in routing section.
imports: [ UniversalModule, RouterModule.forRoot([ { path: '', redirectTo: 'home', pathMatch: 'full' }, { path: 'home', component: HomeComponent }, { path: 'counter', component: CounterComponent }, { path: 'fetch-data', component: FetchDataComponent }, { path: 'hello', component: HelloWorldComponent }, { path: '**', redirectTo: 'home' } ]) ]
Incidentally, the last route (**) means that any unrecognised url (e.g. /nothingmuchimparticular) will redirect to the home page.
Now test it out by running your site and changing the url to end with /hello.
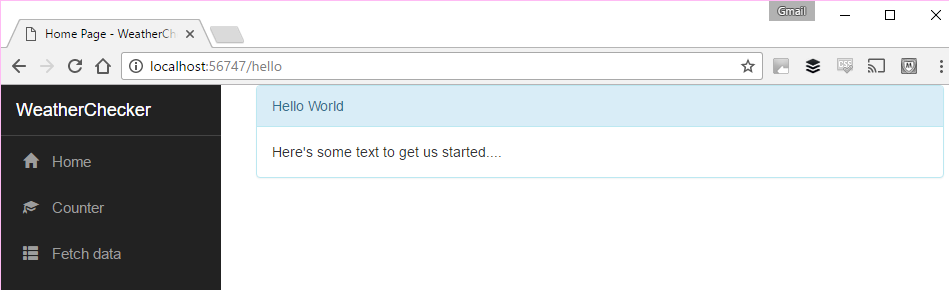
You might be wondering how angular decides where to render the component’s html? It simply looks for the router-outlet
selector and renders your component there.
In our case, the router-outlet tag is included in the main app component (app.component.html).
<div class='container-fluid'> <div class='row'> <div class='col-sm-3'> <nav-menu></nav-menu> </div> <div class='col-sm-9 body-content'> <router-outlet></router-outlet> </div> </div></div>
Link to your route#
If you want to display a link to your new route you’ll need to modify your site’s navigation. As with everything else, the nav menu is its own component.
Modify navmenu.component.html
as follows.
<li [routerLinkActive]="['link-active']"> <a [routerLink]="['/fetch-data']"> <span class='glyphicon glyphicon-th-list'></span> Fetch data </a></li><li [routerLinkActive]="['link-active']"> <a [routerLink]="['/hello']"> <span class='glyphicon glyphicon-user'></span> Hello </a></li>
Now your users can navigate to your friendly greeting…
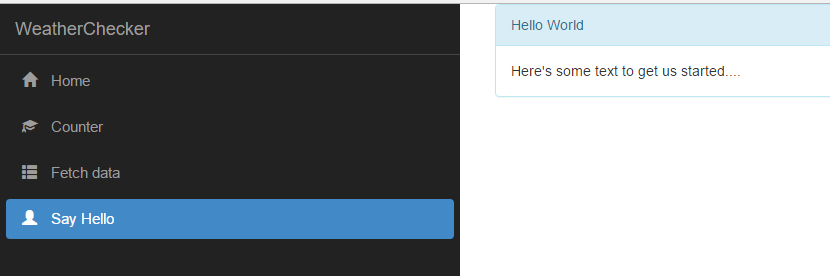
Rain rain, go away…#
Enough HelloWorld, next time we’ll get on with building a more useful component.
We’re going to create a simple component that lets you enter a city name and then see the current weather for that city.
To save storing this data ourselves we’ll use a third party service and connect to it via Web API. That means we’ll go from Angular 2 (our SPA) to .NET Core Web API, which will in turn access the third party weather service.
photo credit: Bill Herndon Farm Road 2775 via photopin (license)
All posts in Checking the weather with Angular 2 and ASP.NET Core
- Fast track your Angular 2 and .NET Core web app development
- Angular 2 and .NET Core – route directly to your components
- Display the weather using Angular 2 and .NET Core Web API
- Angular 2 and .NET Core – your first component
- Fetch the current weather using ASP.NET Core Web API and OpenWeather
- Send form input via an Angular 2 component to ASP.NET Core Web API